After every live stream, I usually go over to the Streamersonglist site and hit the History tab, then manually configure it so it displays all of the songs I played, and in the right order, then manually copy and paste this into the video description in YouTube. More manual cleaning is then needed, to get rid of the unwanted bits of data, leaving just a neat list of song titles and the artists.
It takes a few minutes each time and it’s very repetitive and I’d rather not do it! But I do like to add my set list info to my Youtube recordings as it makes finding performances of certain songs a whole lot easier in future.
Automating the export of your Setlist, right after every stream.
I’m no coder, but I’ve started using the power of ChatGpt to write Python scripts to pull data from sites using their API’s.
I know, it sounds like techno-babble, and it is.
But bear with me – this is actually really easy. If you regularly find yourself wanting to export your set list from Streamersonglist, this will make life easier for you.
Step by step guide
1. You need Python on your machine to run this script
The script uses the Streamersonglist API to retrieve data from your song history, and then creates a simple, clean text file for you with the songs, in the order they were played.
If you don’t already have Python installed, download it for free from Python.org
IMPORTANT: When you install Python, you MUST manually tick the box that says “Add python.exe to PATH
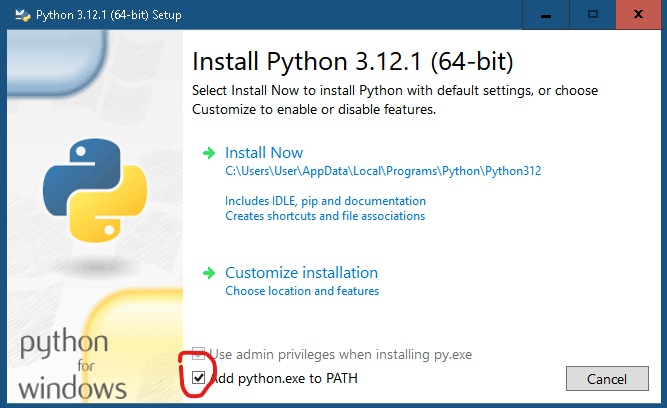
Next, we need to install a module called REQUESTS.
If you’re on Windows, just type CMD in your search bar to open a command prompt and type:
python -m pip install requests
You can find instructions for Windows, Linux and MacOS over at GeeksforGeeks.org
2. Download my little python script
This is a zip file that contains the script.
Extract the file called Setlist.py and copy it to a folder on your computer where the script will run from, and where the set list files will be created.
I have created a folder on my C drive called “python”, and it contains a sub-folder called “setlists”, so my file is located in C:/python/setlists
You will need to be aware of the location of your file, wherever you decide to put it.
3. Edit the script with your own ID number
To find your streamersonglist ID, log in to the site, then navigate to your History page. Right click anywhere on the page and select the “Inspect” option, then click the “Network” tab.
Now reload/refresh the page and look for the line that starts playHistory?size=10¤t=0&type= etc
Mouse over this, and you should see a URL api.streamersonglist.com/v1/streamers/12345/playHistory?size=10¤t…….
Yep, you guessed it, the 12345 in my example, is your unique ID.
You MUST edit the python script and replace the 12345 with your own unique ID.
You should be able to edit the python file with notepad or a similar text or code editor, just right click the file, then open with > notepad (or whatever you prefer).
3. Run the script
You can do this manually using a command prompt, or there are ways to automate it.
In the Windows search bar in the bottom left, type CMD. Then open the Command Prompt.
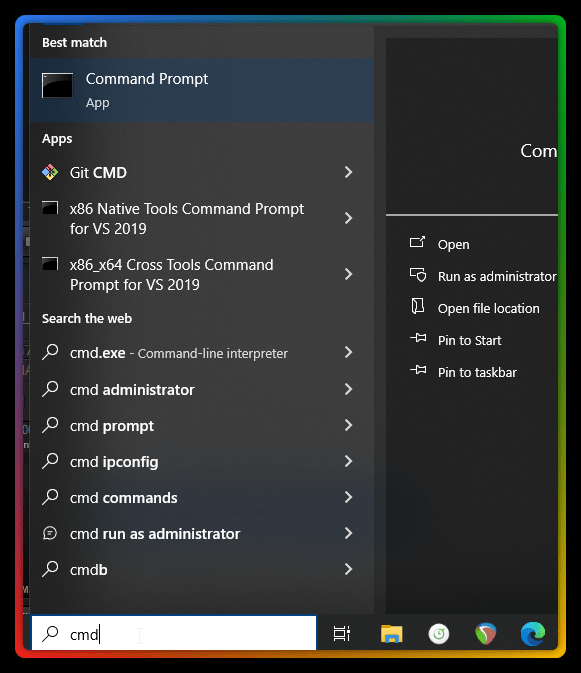
Type cd c:/python/setlist (this needs to be the folder where you saved the python file) then type python setlist.py to run the file.
There are probably a few ways to achieve this. I use TouchPortal, though you could also do it with Streamerbot too.
First create a text file with the following lines:
#Amend to your actual path to the python file
cd c:/python/setlist
python ssl.py
Rename the file so that it ends with .bat instead of .txt
This is a batch file, which you can run from Touchportal or Streamerbot.
In TouchPortal, or Streamerbot, set up a button or action to run the batch file you just created. You could have this triggered when the stream ends, or just add the action to another sequence you might already use at the end of the stream, such as sharing your raid calls, or switching to a “stream ending” scene.
That’s it! The script will create a plain text file for you, which will have the date at the top, followed by a list of the songs you played (and the artist), in order. The filename will also be datestamped.
It’s now quick and easy to copy/paste your set list to wherever it needs to be, e.g. Your youtube VODS.
The script (made with ChatGpt)
If you’d prefer to just copy/paste the code yourself, here it is…
import requests
from datetime import datetime
#You MUST replace the 12345 in the next line with your own unique ID number
# Function to fetch set list from StreamerSongList
def fetch_set_list():
url = "https://api.streamersonglist.com/v1/streamers/12345/playHistory"
params = {
"size": "50",
"current": "0",
"type": "playedAt",
"order": "desc",
"period": "stream",
"songId": "null"
}
response = requests.get(url, params=params)
if response.status_code == 200:
set_list = response.json().get('items', []) # Extracting the 'items' key with default empty list
cleaned_data = []
for entry in set_list:
if 'song' in entry: # Regular song entry
song_info = entry['song']
title = song_info.get('title', 'Unknown Title') # Default to 'Unknown Title' if title is missing
artist = song_info.get('artist', 'Unknown Artist') # Default to 'Unknown Artist' if artist is missing
else: # Non-list song entry
title = entry.get('nonlistSong', 'Unknown Title')
artist = 'Non-Listed' # You can change this as per your preference
cleaned_data.append((title, artist))
# Reverse the order of the songs
cleaned_data.reverse()
# Writing cleaned data to a text file
timestamp = datetime.now().strftime("%Y-%m-%d_%H-%M-%S")
filename = f"set_list_{timestamp}.txt"
with open(filename, "w") as file:
# Write the date at the top of the file
file.write(f"Date: {set_list[0]['playedAt'][:10]}\n\n")
# Write songs without repeating the date
for entry in cleaned_data:
file.write(f"{entry[0]} - {entry[1]}\n")
print("Set list saved to:", filename)
return filename
else:
print("Failed to retrieve set list. Status code:", response.status_code)
return None
# Main function
def main():
# Fetch set list from StreamerSongList
file_setlist = fetch_set_list()
if not file_setlist:
return
if __name__ == '__main__':
main()
Change the “size” parameter to alter the maximum number of songs to retrieve. If you set this figure to a number greater than the number of songs in your history, it can cause problems, so don’t do that.
# Note: you can also modify the script to search over a wider period of time by changing the “period” from “stream” to “all”, “day”, “week”, “month” – basically any of the options you see on the History page on the website.
Notes: the script didn’t work if a non-list song was played. This has been corrected so the output now also includes non-list songs.
Leave a Reply